Simple JSON API using Express
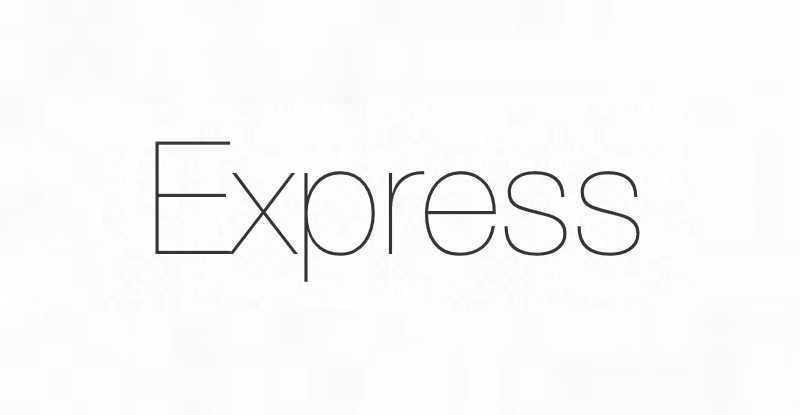
Express is a very popular web framework for Node JS which is a javascript runtime built on Chrome's V8 javascript engine. Node can run on Windows, Mac or Linux and so can be used on your web server.
If you are new to Express, this is for you. Basic knowledge of Node and requiring node packages in your code is required.
Before we can get into programming the API itself, first we need to make a project folder and initialize package.json
file so we can install the Express framework.
Make a new directory for your example API and change into it:
mkdir ExampleAPI
cd ExampleAPI
Create a package.json
file with no questions asked and install the express framework
npm init -y
npm i express compression cors helmet body-parser
After a small download you should now see a new folder in your project directory called node_modules
this is where the node package manager stores your project specific dependencies.
Note: If you are using source control, you should update your
.gitignore
file to ignore thisnode_modules
folder. If you clone this on another machine, to install the packages again you just typenpm i
in the project directory.
We have installed the express framework itself, along with some popular middleware that helps with security performace and parsing requests.
Express has a simple hello world example on their website, that we will use to get up and running with our extremely simple example API quickly. We are going to modify it slightly in order to use some middleware and return a JSON response. We'll also add a simple echoing endpoint for post requests so our API behaves much like other API's you might be familiar with.
Making a more interesting API is a challenge I leave to you.
index.js:
// Require all the dependencies
const express = require('express');
const compression = require('compression');
const cors = require('cors');
const helmet = require('helmet');
const bodyParser = require('body-parser');
// Create the app
const app = express();
const port = 3000;
// Rudimentary logging
function log(message) {
console.log(`[${new Date().toISOString()}] ${message}`);
}
// Add middleware to the express app
app.use(cors());
app.use(helmet());
app.use(compression());
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json({ extended: true }));
// Routes are registered prior to starting the server
// for more info on how routing works see the docs
// https://expressjs.com/en/guide/routing.html
// Simple route for displaying a messing on the root route
app.get('/', (req, res) => {
log(`GET /`);
res.status(200).json({ message: 'Hello World' });
});
// Echo back any data provided under the 'message' key
app.post('/echo', (req, res) => {
log(`POST /echo -> ${JSON.stringify(req.body)}`);
res.status(200).json({ message: req.body.message });
});
// Start the server
app.listen(port, () => {
log(`Example JSON API listening at http://localhost:${port}`);
});
With that done, we can test our new API by starting up the app from the terminal:
node index.js
# Example app listening at http://localhost:3000
Using curl
in a new terminal window you can test the response from the root route
curl http://localhost:3000
# {"message":"Hello World"}
And also we can POST
some JSON data to our echo endpoint:
curl --header "Content-Type: application/json" \
--request POST \
--data '{ "message": "This is my new JSON API" }' \
http://localhost:3000/echo
# {"message":"This is my new JSON API"}